There’s a great article by Yegor Bugayenko called Need Robust Software? Make It Fragile. It’s about Fail Fast philosophy where you should write your code in the way that your application will fail as fast as possible if something is significantly wrong with your data or environment. When you’re building a mobile game with Unity then most probably you’re […]
Unity 5.1 Assertion Library
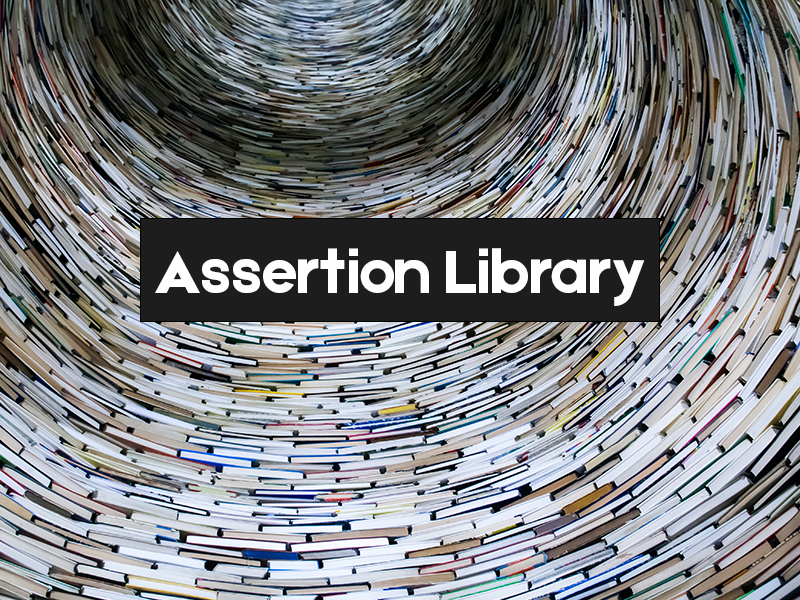